Lists, a versatile and fundamental data structure in Python, play a pivotal role in various programming scenarios. In this comprehensive guide, we will explore the creation, manipulation, and advanced features of lists in Python.
Understanding Lists
A list is an ordered collection of elements enclosed in square brackets [ ]
and separated by commas. Python allows lists to contain elements of different types, including integers, floats, strings, and even other lists.
Let’s start by creating a simple list:
# Creating a basic list cogxta_list = ["Python", "Anaconda", "Jupyter"] print(cogxta_list)
This yields the output:
['Python', 'Anaconda', 'Jupyter']
Diving Deeper: Mixed Data Types and Nested Lists
Lists in Python are incredibly flexible, supporting mixed data types and even nested structures. Consider the following examples:
# List with mixed data types mixed_list = [5, "Python", 11.3]
print(mixed_list)
Output:
[5, 'Python', 11.3]
Additionally, Python allows lists to be nested, meaning a list can contain another list:
# Nested list nested_list = ["Anaconda", [11.3, 5, 1], ['cogxta']] print(nested_list)
Output:
['Anaconda', [11.3, 5, 1], ['cogxta']]
Accessing List Elements
Python lists are zero-indexed, meaning the first element is at index 0, the second at index 1, and so forth. You can access list elements using square brackets and the index:
# Accessing list elements
cogxta_list = ['p', 'y', 't', 'h', 'o', 'n'] #
First item print(cogxta_list[0]) # Output: p
# Third item print(cogxta_list[2])
# Output: t
# Fifth item print(cogxta_list[4])
# Output: o
For nested lists, you can use nested indexing:
# Nested indexing nested_list = ["Anaconda", [2, 0, 1, 5]]
print(nested_list[0][1])
# Output: n print(nested_list[1][3])
# Output: 5
Attempting to use non-integer values for indexing will result in a TypeError
.
Mastering List Slicing
List slicing allows you to extract a range of elements from a list. The syntax involves specifying the start and end indices separated by a colon :
:
# List slicing cogxta_list = ['p', 'y', 't', 'h', 'o', 'n']
# Elements from index 2 to index 4 print(cogxta_list[2:5])
# Output: ['t', 'h', 'o']
# Elements from index 5 to the end print(cogxta_list[5:])
# Output: ['n']
# All elements (beginning to end) print(cogxta_list[:])
# Output: ['p', 'y', 't', 'h', 'o', 'n']
Constructing Lists with list() Constructor
Python provides the list()
constructor to create a list. This constructor can convert other iterable objects, like tuples or strings, into lists:
# Using list() constructor
cogxta_list = list(("Python", "Anaconda", "Jupyter"))
print(cogxta_list)
Output:
['Python', 'Anaconda', 'Jupyter']
Unveiling Powerful List Methods
Python’s list class comes equipped with a variety of methods, making list manipulation a breeze. Here are some frequently used methods:
- append(): Adds an element to the end of the list.
- extend(): Adds all elements of one list to another list.
- insert(): Inserts an item at a specified index.
- remove(): Removes an item from the list.
- pop(): Returns and removes an element at the given index.
- clear(): Removes all items from the list.
- index(): Returns the index of the first matched item.
- count(): Returns the count of items passed as an argument.
- sort(): Sorts items in ascending order.
- reverse(): Reverses the order of items in the list.
- copy(): Returns a shallow copy of the list.
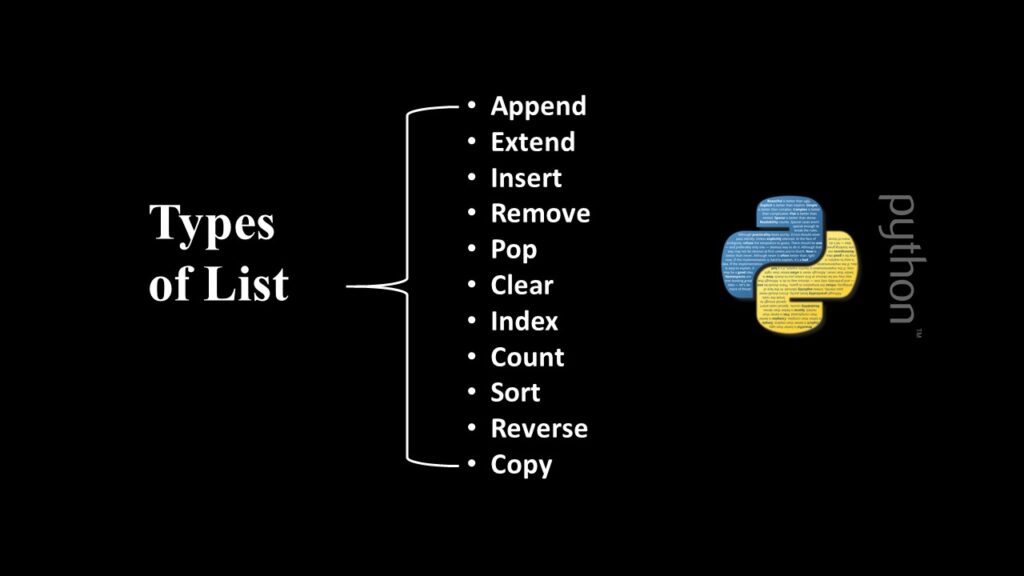
# Example of list methods
cogxta_list = [1, 2, 3]
# Append an element cogxta_list.append(4)
# Extend the list cogxta_list.extend([5, 6])
# Insert an element at index 2 cogxta_list.insert(2, 10)
# Remove the element with value 3 cogxta_list.remove(3)
# Pop the element at index 1 cogxta_list.pop(1)
# Sort the list cogxta_list.sort()
# Reverse the list cogxta_list.reverse()
# Copy the list copied_list = cogxta_list.copy()
Embracing List Comprehension
List comprehension is a concise and elegant method for creating lists in Python. It involves specifying an expression followed by a for statement inside square brackets. Consider the following example:
# List comprehension example
cog = [x for x in range(10)]
print(cog)
Output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
This is equivalent to the traditional for loop approach:
# Equivalent using a for loop cog = []
for x in range(10):
cog.append(x)
print(cog)
Output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
In summary, mastering lists in Python involves understanding their creation, accessing elements, utilizing slicing, employing the list() constructor, leveraging powerful methods, and embracing the elegance of list comprehension. Lists are a foundational concept in Python, and proficiency in working with them is key to becoming a proficient Python programmer. Whether you are a beginner or an experienced developer, harnessing the power of lists will undoubtedly enhance your Python programming skills.
Leave a Reply