Dictionaries in Python are powerful data structures that allow you to store key-value pairs. Often, there arises a need to sort a dictionary based on its values. In this exploration, we’ll uncover the techniques to efficiently sort a dictionary in both ascending and descending order.
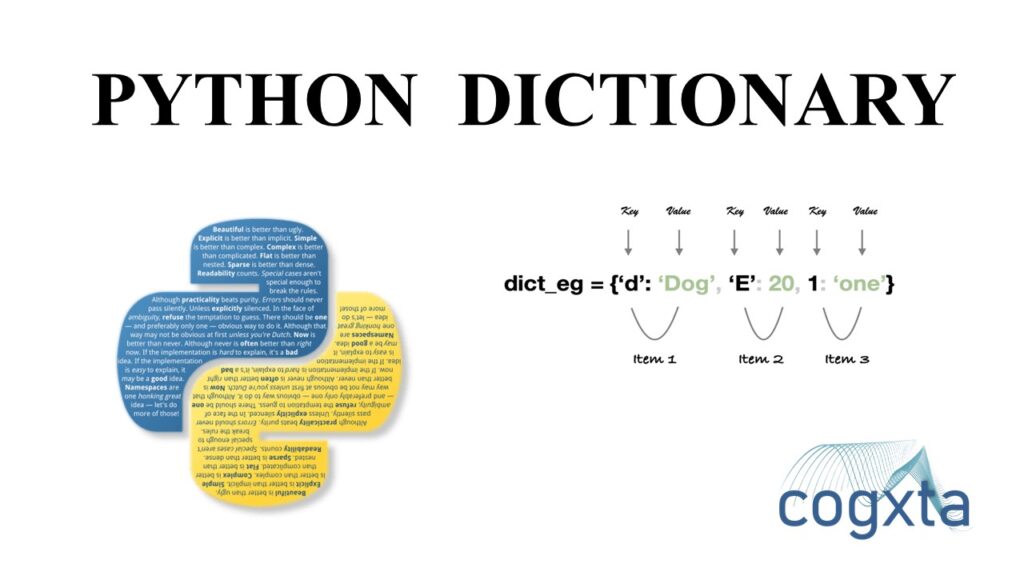
Example Dictionary Object
Let’s consider a sample dictionary to demonstrate the sorting techniques.
my_dictionary = {'b': 4, 'c': 6, 'a': 3, 'd': 2} print(my_dictionary)
Output:
{'b': 4, 'c': 6, 'a': 3, 'd': 2}
Sort in Ascending Order
To sort the dictionary in ascending order based on values, the sorted()
function can be utilized with a lambda function as the key parameter.
sorted_dict_asc = sorted(my_dictionary.items(), key=lambda i: i[1], reverse=False) print(sorted_dict_asc)
Output:
[('d', 2), ('a', 3), ('b', 4), ('c', 6)]
Sort in Descending Order
Similarly, for descending order, the sorted()
function can be employed with the reverse
parameter set to True
.
sorted_dict_dsc = sorted(my_dictionary.items(), key=lambda i: i[1], reverse=True) print(sorted_dict_dsc)
Output:
[('c', 6), ('b', 4), ('a', 3), ('d', 2)]
Reformatting to Dictionary
The sorted results are obtained as a list of tuples. To convert them back to a dictionary, a simple loop can be employed.
new_dict = {} for k, v in sorted_dict_dsc: new_dict[k] = v print(new_dict)
Output:
{'c': 6, 'b': 4, 'a': 3, 'd': 2}
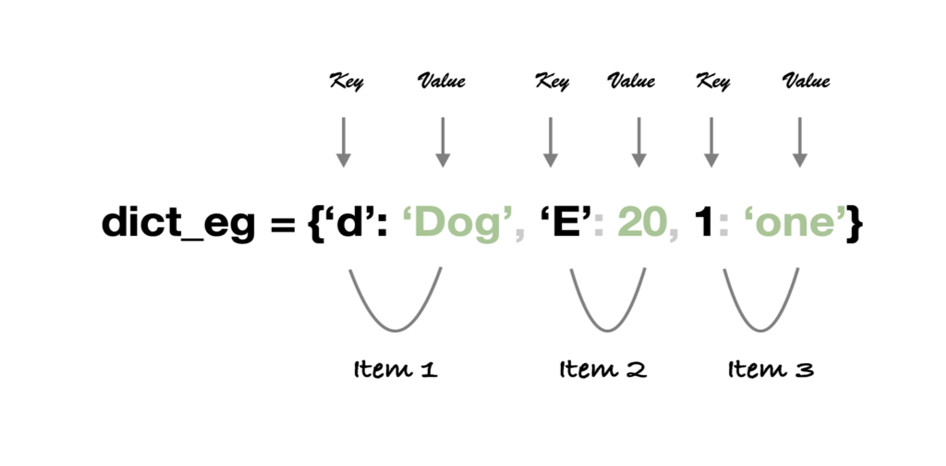
Conclusion
In conclusion, sorting dictionaries in Python is a straightforward process using the sorted()
function and lambda functions to specify the key for sorting. Whether you need your dictionary sorted in ascending or descending order, these techniques provide an efficient solution to meet your requirements. Mastering these skills will empower you to handle and manipulate dictionaries effectively in various Python applications.
Leave a Reply