Exploratory Data Analysis (EDA)
Exploratory Data Analysis (EDA) is a crucial step in understanding and preparing data for building predictive models. In this lab, we focus on visualizing the dataset related to automobile pricing using Python. The dataset is loaded and cleaned, and now we’ll explore it through various visualizations.
Summarizing and Manipulating Data:
- Understand the size of the dataset.
- Identify interesting columns.
- Derive characteristics of the data using summary statistics and counts.
Developing Multiple Views of Complex Data:
- Utilize multiple chart types for exploring complex data.
- Understand the importance of various visualizations in gaining a comprehensive understanding.
Overview of Plotting Packages:
- Introduction to Matplotlib, Pandas plotting, and Seaborn.
Univariate and Bivariate Plot Types:
- Review of basic plot types using three Python packages to study distributional properties and relationships between two variables.
Using Aesthetics:
- Overview of projecting additional plot dimensions using plot aesthetics.
Facetted Plotting:
- Introduction to a powerful method for visualizing higher-dimensional data, arranging arrays of plots on the 2D computer graphics display.
Adding Attributes with Matplotlib:
- Using Matplotlib methods to add attributes like titles and axis labels to plots.
Summary of the Dataset
Let’s begin by summarizing the dataset. The columns include information such as make, fuel type, body style, horsepower, and price. Before diving into more advanced visualizations, let’s understand the distribution of some key features.
# Summary Statistics
summary_stats = auto_prices.describe()
# Count of Unique Values in Categorical Columns
unique_counts = auto_prices.nunique()
# Visualizing Missing Values
plt.figure(figsize=(10, 6))
sns.heatmap(auto_prices.isnull(), cbar=False, cmap='viridis')
plt.title('Missing Values in the Dataset')
plt.show()
The summary statistics provide insights into numerical features, and the heatmap visually indicates missing values in the dataset.
Univariate Visualizations
Now, let’s explore the distribution of individual features. We’ll use histograms to visualize the distribution of numeric variables.
# Univariate Visualization: Histograms
num_cols = auto_prices.select_dtypes(include=['int64', 'float64']).columns
auto_prices[num_cols].hist(bins=20, figsize=(15, 12))
plt.suptitle('Distribution of Numeric Variables')
plt.show()
Histograms provide a quick overview of the distribution of numerical variables like wheel-base, length, width, etc.
Bivariate Visualizations
Moving on to relationships between variables, scatter plots are a common choice. Let’s create scatter plots for some pairs of variables.
# Bivariate Visualization: Scatter Plots
sns.pairplot(auto_prices[['wheel-base', 'length', 'width', 'curb-weight', 'engine-size', 'horsepower', 'price']])
plt.suptitle('Pairwise Relationships')
plt.show()
The pairplot displays scatter plots for selected variables, helping us identify potential relationships.
Correlation Heatmap
Correlation heatmaps are valuable for understanding relationships between numeric variables.
# Correlation Heatmap
correlation_matrix = auto_prices.corr()
plt.figure(figsize=(12, 10))
sns.heatmap(correlation_matrix, annot=True, cmap='coolwarm', fmt='.2f')
plt.title('Correlation Heatmap')
plt.show()
This heatmap illustrates the correlation between different features, with values closer to 1 indicating a stronger correlation.
Box Plots
Box plots can reveal the distribution of a numeric variable for each category of a categorical variable.
# Box Plots
plt.figure(figsize=(14, 8))
sns.boxplot(x='body-style', y='price', data=auto_prices)
plt.title('Price Distribution by Body Style')
plt.show()
Box plots help visualize the spread and central tendency of prices based on different body styles.
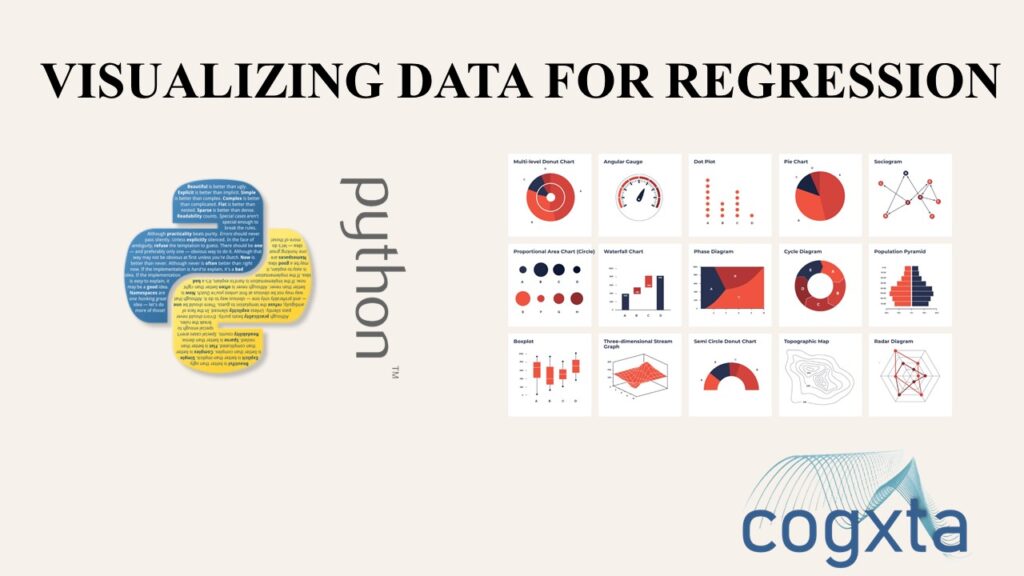
These visualizations provide an initial understanding of the dataset’s characteristics, distributions, and relationships. Further analysis and feature engineering can be performed based on these insights. Remember, the specific visualizations and analyses depend on the dataset and the objectives of the regression analysis.
In subsequent labs, we’ll delve deeper into preparing data and building regression models. Stay tuned for more insights into predictive modeling with Python!
Leave a Reply