Practical Applications and Advanced Concepts
Introduction:
Welcome to the third and final part of our tutorial on Image Processing and Object Comparison using Python. In this section, we’ll explore practical applications and advanced concepts that build upon the knowledge gained in the previous parts. By the end of this tutorial, you’ll be equipped with the skills to tackle real-world image processing challenges.
Practical Application: Image Alignment and Feature Matching:
One common application of image processing is aligning images and identifying corresponding features. This is useful in tasks like panoramic image stitching. Let’s use the popular OpenCV library to align two images and find matching features.
import cv2
# Load images
img1 = cv2.imread(outloc + "hline.jpg", cv2.IMREAD_GRAYSCALE)
img2 = cv2.imread(outloc + "rotate.jpg", cv2.IMREAD_GRAYSCALE)
# Create ORB detector
orb = cv2.ORB_create()
# Find key points and descriptors
kp1, des1 = orb.detectAndCompute(img1, None)
kp2, des2 = orb.detectAndCompute(img2, None)
# Create BFMatcher (Brute Force Matcher)
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# Match descriptors
matches = bf.match(des1, des2)
# Sort them based on distance
matches = sorted(matches, key=lambda x: x.distance)
# Draw matches
img_matches = cv2.drawMatches(img1, kp1, img2, kp2, matches[:10], None, flags=cv2.DrawMatchesFlags_NOT_DRAW_SINGLE_POINTS)
# Display the result
plt.imshow(img_matches)
plt.title("Feature Matching")
plt.show()
Advanced Concept: Convolutional Neural Networks (CNNs) for Image Comparison:
For more advanced scenarios, Convolutional Neural Networks (CNNs) can be employed. Let’s use a pre-trained CNN model for image comparison using the popular TensorFlow and Keras libraries.
from tensorflow.keras.applications import VGG16
from tensorflow.keras.preprocessing import image
from tensorflow.keras.applications.vgg16 import preprocess_input, decode_predictions
# Load the VGG16 model pre-trained on ImageNet data
model = VGG16(weights='imagenet', include_top=False)
# Load and preprocess images
img1 = image.load_img(outloc + "hline.jpg", target_size=(224, 224))
img2 = image.load_img(outloc + "rotate.jpg", target_size=(224, 224))
img1_array = image.img_to_array(img1)
img2_array = image.img_to_array(img2)
img1_array = np.expand_dims(img1_array, axis=0)
img2_array = np.expand_dims(img2_array, axis=0)
img1_array = preprocess_input(img1_array)
img2_array = preprocess_input(img2_array)
# Get the CNN features
features1 = model.predict(img1_array)
features2 = model.predict(img2_array)
# Calculate cosine similarity between features
cosine_similarity = cosin_sim(features1.flatten(), features2.flatten())
print(f"Cosine Similarity using CNN Features: {cosine_similarity}")
Conclusion:
In this final part, we applied image alignment and feature matching techniques using OpenCV and explored the advanced concept of using Convolutional Neural Networks (CNNs) for image comparison. These techniques are instrumental in real-world scenarios, from creating panoramic images to leveraging the power of deep learning for complex image analysis.
With the knowledge gained from this tutorial, you are well-equipped to handle various image processing tasks and apply these techniques to your projects.
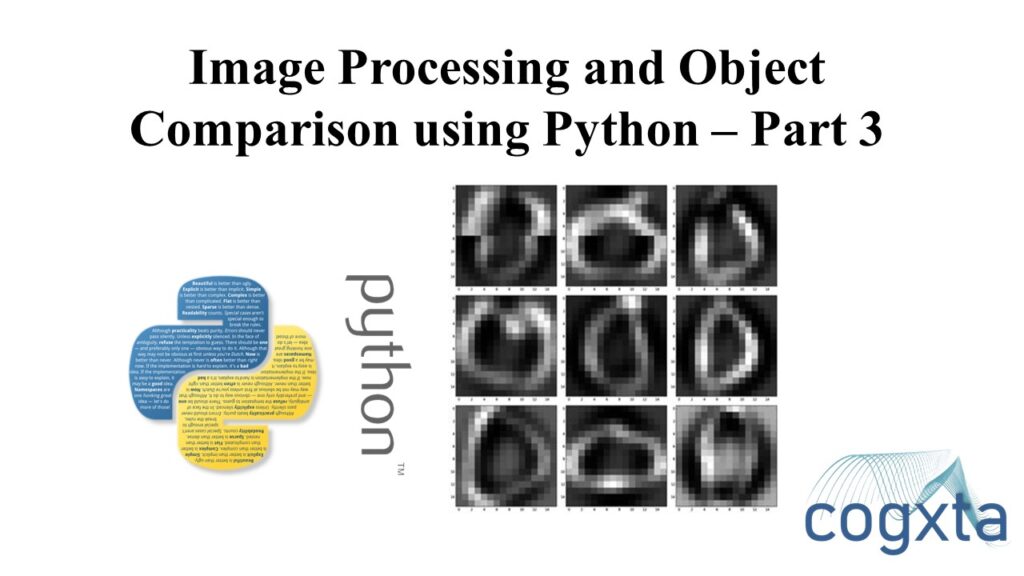
Thank you for joining us on this journey into Image Processing and Object Comparison using Python!
Leave a Reply