Numpy, Your Gateway to Powerful Array Manipulation in Python
If you’re venturing into the realm of scientific computing or data analysis with Python, Numpy is your trusted companion. This library is tailored for multidimensional array operations, offering features like seamless data consistency checks, efficient memory usage, and lightning-fast vector arithmetic. In this comprehensive guide, we’ll unravel the essentials of Numpy, from basic array initialization to advanced operations and statistical functions.
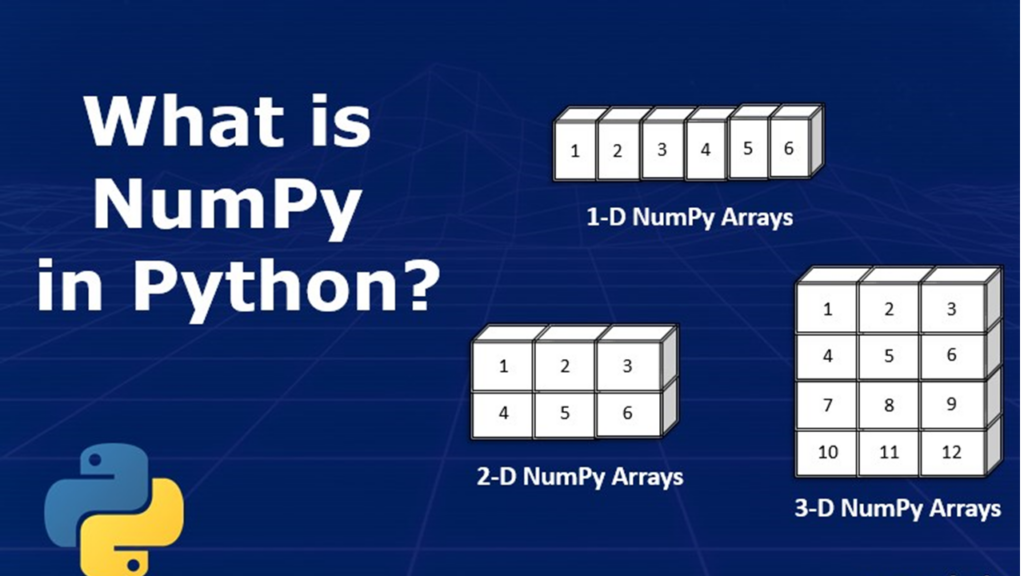
Introduction to Numpy
Numpy’s strength lies in its ability to manage multidimensional arrays effortlessly. Let’s kick off our journey by understanding its key features.
# Importing Numpy
import numpy as np
Initialization of 1D Array in Python
Numpy arrays introduce crucial state variables – dtype
and shape
. Here are two methods to initialize a 1D array.
# Initializing from Python List
v = np.array([1, 4, 9, 3, 2])
print('1D array in numpy is %s\n' % v)
print('dtype of the numpy array is %s\n' % str(v.dtype))
print('shape of the numpy array is %s\n' % v.shape)
Initialization of 2D Array in Python
Understanding the row-major format is essential for 2D arrays in Numpy. Learn about initialization from a list of lists and other methods.
# Initialization from List of List
pymat = [[1, 2, 3], [4, 5, 6]]
npmat = np.array(pymat) print('numpy matrix is \n%s\n' % npmat)
print('Flattened version of numpy matrix is %s\n' % npmat.flatten())
Slicing and Indexing in Numpy
Explore various slicing techniques for 1D arrays. Discover how to access elements, remove elements, and create subarrays.
# Remove n elements vec1 = vec[:-3]
print('Result of removing the last 3 elements from range(10): \n%s\n' % vec1)
# Access elements at even indices in a 1D array vec2 = vec[::2]
print('Elements at even indices are %s\n' % vec2)
# Access elements at indices in reverse order
vec2 = vec[::-1]
print('Elements for indices in reverse are %s\n' % vec2)
Unleashing Advanced Numpy Operations
Now that we’ve grasped the basics, let’s dive into advanced operations and features that make Numpy a powerhouse for array manipulation.
Understanding – Pass By Reference
Explore the pass-by-reference behavior of Numpy arrays. Understand how modifications in one array can affect another.
mat1 = np.random.random((2, 3))
pt1 = mat1[0].__array_interface__['data'][0]
pt2 = mat1[1].__array_interface__['data'][0]
print('Memory Location of mat1[0] is: %s\n' % pt1)
print('Memory Location of mat1[1] is: %s\n' % pt2)
print('Difference in Memory Location for 3 elements is: %s bytes\n' % (pt2 - pt1))
Broadcast Operation in Numpy
Understand the concept of broadcasting, a powerful feature for element-wise operations on arrays with different shapes.
mat = np.random.randint(0, 6, (3, 5))
v = np.array([10, 20, 30, 40, 50])
print('mat + v is \n%s\n' % (mat + v))
print('mat * v is \n%s\n' % (mat * v))
Matrix Operations
Learn about inner and outer products in Numpy, including vector-vector, matrix-vector, and matrix-matrix operations.
print('Matrix Vector product is <mat, vec1> is \n%s\n' % np.dot(mat, vec1)) print('Matrix Matrix multiplication is <mat, mat> is \n%s\n' % np.dot(mat, mat))
Statistical Functions
Explore statistical functions provided by Numpy, such as mean, variance, sum, min, max, and percentile.
marks = np.array([30, 31, 32, 40, 90, 95, 97, 98, 99, 100])
print('Overall 30 percent quantile of marks is %s\n' % (str(np.percentile(marks, 30))))
Miscellaneous Functions
Discover useful functions like squeeze
and transpose
for reshaping and transforming arrays.
# Squeeze example
ndmat = np.zeros(shape=(1, 2, 3, 1))
print('Original Shape of ndmat is %s\n' % str(ndmat.shape))
ndmat1 = np.squeeze(ndmat)
print('Shape of np.squeeze(ndmat) is %s\n' % str(ndmat1.shape))
# Transpose example
mat = np.zeros(shape=(2, 3))
print('Original Shape of 2D matrix is %s\n' % str(mat.shape))
mat_transpose = np.transpose(mat)
print('Shape of np.transpose(mat) is %s\n' % str(mat_transpose.shape))
Conclusion
These comprehensive blog posts serve as your go-to guide for mastering Numpy, covering everything from array initialization to advanced operations and statistical functions. Unlock the full potential of Numpy in your Python journey!
Leave a Reply