In Python, a tuple is a versatile data structure that allows you to store ordered and immutable sequences of elements. In this exploration, we’ll delve into the characteristics, operations, and manipulation techniques associated with tuples.
Understanding Tuples
A tuple is defined by enclosing a sequence of Python objects in round brackets. It is comparable to a list in terms of indexing, nested objects, and repetition. However, a key distinction lies in its immutability โ once a tuple is created, its elements cannot be changed, added, or removed.
Quick Example
Let’s start with a quick example to illustrate the creation of a tuple.
cogxta_Tup = ("Python", "Anaconda", "jupyter")
print(cogxta_Tup)
Output:
('Python', 'Anaconda', 'jupyter')
Tuple Characteristics
Tuple Items
Tuple items are immutable, sorted, and allow for duplicate values. The indexing starts at [0], and elements can be accessed using positive or negative indexing.
cogxta_Tup = ("Python", "Anaconda", "jupyter")
print(cogxta_Tup[1]) # Accessing the second item
Output:
Anaconda
Range of Indexes
You can specify a range of indexes to retrieve a subset of elements from a tuple.
cogxta_Tup = ("ant", "badger", "cat", "dog", "elephant", "frog", "giraffe") print(cogxta_Tup[2:5]) # Retrieving items from index 2 to 4
Output:
('cat', 'dog', 'elephant')
Unpack Tuples
Values from a tuple can be extracted into variables, a process known as “unpacking.”
cogxta_Tup = ("ant", "badger", "cat") (red, brown, black) = cogxta_Tup
print(red)
print(brown)
print(black)
Output:
ant badger cat
Manipulating Tuples
Change Tuple Values
Although tuples are immutable, you can achieve a similar effect by converting a tuple to a list, making the necessary changes, and converting it back to a tuple.
cogxta_Tup_x = ("ant", "badger", "cat") cogxta_Tup_y = list(cogxta_Tup_x) cogxta_Tup_y[1] = "dog" cogxta_Tup_x = tuple(cogxta_Tup_y)
print(cogxta_Tup_x)
Output:
('ant', 'dog', 'cat')
Add Items
To add items to a tuple, you can either convert it to a list, add the item(s), and convert it back to a tuple or create a new tuple and concatenate it with the existing one.
# Convert to a list and back
cogxta_Tup = ("ant", "badger", "cat")
cogxta_y = list(cogxta_Tup)
cogxta_y.append("frog")
cogxta_Tup = tuple(cogxta_y)
print(cogxta_Tup)
# Create a new tuple and concatenate
cogxta_Tup = ("ant", "badger", "cat")
cogxta_y = ("frog",)
cogxta_Tup += cogxta_y
print(cogxta_Tup)
Output:
('ant', 'badger', 'cat', 'frog') ('ant', 'badger', 'cat', 'frog')
Remove Items
Similarly, you can remove items from a tuple by converting it to a list, making the necessary changes, and converting it back to a tuple.
cogxta_Tup = ("ant", "badger", "cat")
cogxta_y = list(cogxta_Tup)
cogxta_y.remove("ant")
cogxta_Tup = tuple(cogxta_y)
print(cogxta_Tup)
Output:
('badger', 'cat')
Delete Tuple
The entire tuple can be deleted using the del
keyword.
cogxta_Tup = ("ant", "badger", "cat")
del cogxta_Tup
Attempting to print cogxta_Tup
after deletion will result in a NameError
since the tuple no longer exists.
Advanced Tuple Operations
Using Asterisk (*)
Asterisk can be used for unpacking and handling variable numbers of elements.
cogxta_Tup = ("ant", "badger", "cat", "dog", "elephant", "frog", "giraffe")
(red, *brown, black) = cogxta_Tup
print(red)
print(brown)
print(black)
Output:
ant ['badger', 'cat', 'dog', 'elephant', 'frog']
giraffe
Join Two Tuples
Tuples can be joined using the +
or *
operator.
# Join with +
cogxta_tuple1 = ("a", "b", "c")
cogxta_tuple2 = (1, 2, 3)
cogxta_tuple3 = cogxta_tuple1 + cogxta_tuple2
print(cogxta_tuple3)
# Multiply with *
cogxta_Tup = ("ant", "badger", "cat")
new_tuple = cogxta_Tup * 2
print(new_tuple)
Output:
('a', 'b', 'c', 1, 2, 3) ('ant', 'badger', 'cat', 'ant', 'badger', 'cat')
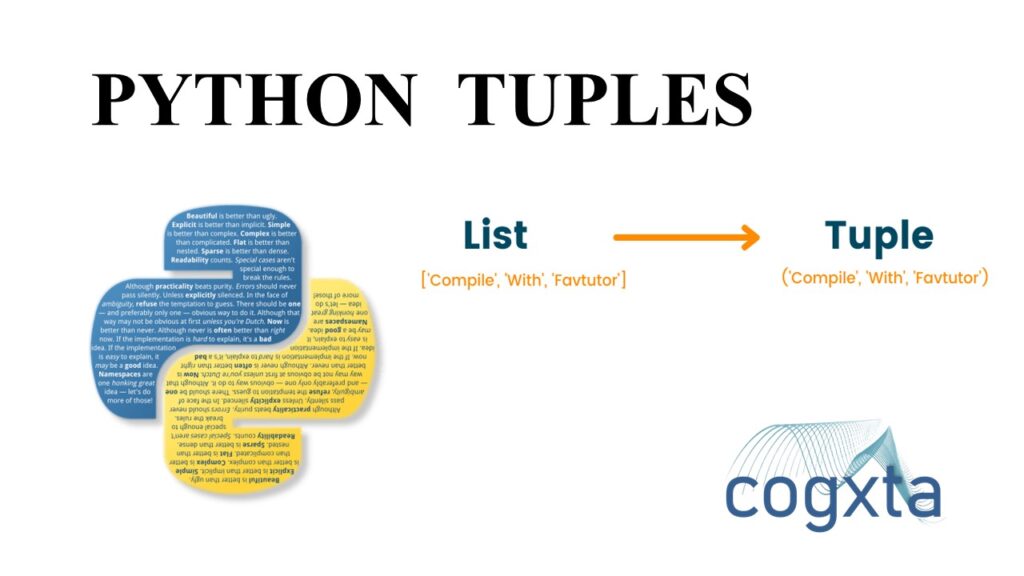
Tuples in Python provide a powerful and flexible way to handle ordered, immutable sequences of elements. Whether you are working with data that should not be changed or need to efficiently manage multiple values, mastering tuples will significantly enhance your Python programming skills.
Leave a Reply