Named Entity Recognition (NER) is a fundamental task in natural language processing that involves identifying and classifying entities, such as names of people, organizations, and locations, within a text. NER plays a crucial role in various applications, including information retrieval, question answering, and text summarization.
In this blog post, we’ll dive into the world of NER and explore how to build a Named Entity Recognition system using Conditional Random Fields (CRFs). CRFs are a type of probabilistic graphical model that has proven effective in sequence labeling tasks, making them particularly well-suited for NER.
Understanding the Dataset
Before delving into the intricacies of CRFs, let’s take a moment to understand the dataset we’ll be working with. We’ll be using the Dutch CoNLL-2002 dataset, which is a collection of sentences annotated with named entities such as persons, organizations, and locations. The dataset is split into training, development, and test sets, providing a robust foundation for training and evaluating our NER system.
# Importing necessary libraries and loading the dataset
import nltk
import sklearn
from sklearn.metrics import classification_report, confusion_matrix
from sklearn.preprocessing import LabelBinarizer
import sklearn_crfsuite as crfsuite
from sklearn_crfsuite import metrics
train_sents = list(nltk.corpus.conll2002.iob_sents('ned.train'))
dev_sents = list(nltk.corpus.conll2002.iob_sents('ned.testa'))
test_sents = list(nltk.corpus.conll2002.iob_sents('ned.testb'))
Feature Engineering with Word Clusters
Feature engineering plays a pivotal role in the success of any machine learning model, and NER with CRFs is no exception. One intriguing aspect of the feature engineering process in this context is the utilization of word clusters. These clusters, derived from pre-trained word embeddings, provide valuable information about the relationships between words in a given text.
# Function to read word clusters from a file
def read_clusters(cluster_file):
word2cluster = {}
with open(cluster_file) as i:
for line in i:
word, cluster = line.strip().split('\t')
word2cluster[word] = cluster
return word2cluster
Leveraging Word Features for NER
Now that we have our dataset and word clusters in place, the next step involves transforming our raw text into a format suitable for training a Conditional Random Field. This entails defining features for each word in our dataset. The word2features
function achieves this by extracting relevant information such as word morphology, part-of-speech tags, and neighboring words.
#Function to extract features for a given word in a sentence
def word2features(sent, i, word2cluster):
word = sent[i][0]
postag = sent[i][1]
features = [
'bias',
'word.lower=' + word.lower(),
'word[-3:]=' + word[-3:],
'word[-2:]=' + word[-2:],
'word.isupper=%s' % word.isupper(),
'word.istitle=%s' % word.istitle(),
'word.isdigit=%s' % word.isdigit(),
'word.cluster=%s' % word2cluster[word.lower()] if word.lower() in word2cluster else "0",
'postag=' + postag
]
# … (continuation)
This function takes a sentence, the index of the current word in the sentence, and the word clusters as input and returns a list of features for that word. Features include basic characteristics of the word, such as its lowercase form, suffixes, capitalization, and more.
The sent2features
function extends this process to the entire sentence, generating a list of feature sets for each word in the sentence.
# Function to convert a sentence to a list of feature sets
def sent2features(sent, word2cluster):
return [word2features(sent, i, word2cluster) for i in range(len(sent))]
]
With the feature extraction in place, we can now transform our dataset into features and labels suitable for training our CRF model.
# Applying feature extraction to the training, development, and test sets
X_train = [sent2features(s, word2cluster) for s in train_sents]
y_train = [sent2labels(s) for s in train_sents]
X_dev = [sent2features(s, word2cluster) for s in dev_sents]
y_dev = [sent2labels(s) for s in dev_sents]
X_test = [sent2features(s, word2cluster) for s in test_sents]
y_test = [sent2labels(s) for s in test_sents]
With our features and labels prepared, we can proceed to train our Conditional Random Field model.
Training the CRF Model
Once the features and labels are prepared, we can proceed with training our CRF model. In this example, we are using the sklearn_crfsuite
library, which provides an implementation of the Conditional Random Field algorithm.
# Creating and training the CRF model
crf = crfsuite.CRF(
verbose='true',
algorithm='lbfgs',
max_iterations=100
)
crf.fit(X_train, y_train, X_dev=X_dev, y_dev=y_dev)
Here, we instantiate the CRF model, specifying some parameters like verbosity, the optimization algorithm (L-BFGS in this case), and the maximum number of iterations. We then fit the model to our training data while also providing a development set for evaluation during training.
Saving and loading the trained model for later use is facilitated using the joblib
library, which serializes Python objects.
# Saving the trained CRF model to a file
import joblib
import os
OUTPUT_PATH = "models/ner/"
OUTPUT_FILE = "crf_model"
if not os.path.exists(OUTPUT_PATH):
os.mkdir(OUTPUT_PATH)
joblib.dump(crf, os.path.join(OUTPUT_PATH, OUTPUT_FILE))
# Loading the trained CRF model from a file
crf = joblib.load(os.path.join(OUTPUT_PATH, OUTPUT_FILE))
Now that our CRF model is trained and loaded, we can proceed with making predictions on the test set and evaluating its performance.
Evaluating the Model
The predict
function is used to obtain predictions for the test set, and these predictions are then flattened for easy comparison with the ground truth labels.
# Making predictions on the test set
y_pred = crf.predict(X_test)
# Flatten the nested lists
y_test_flat = [label for sublist in y_test for label in sublist]
y_pred_flat = [label for sublist in y_pred for label in sublist]
With the predictions and ground truth labels prepared, we can utilize various evaluation metrics to assess the performance of our model.
# Print classification report
print(classification_report(y_test_flat, y_pred_flat, labels=labels))
# Print confusion matrix
conf_matrix = confusion_matrix(y_test_flat, y_pred_flat, labels=labels)
print(conf_matrix)
# Print additional evaluation metrics
print("Precision:", metrics.flat_precision_score(y_test, y_pred, average='weighted'))
print("Recall:", metrics.flat_recall_score(y_test, y_pred, average='weighted'))
print("F1-Score:", metrics.flat_f1_score(y_test, y_pred, average='weighted'))
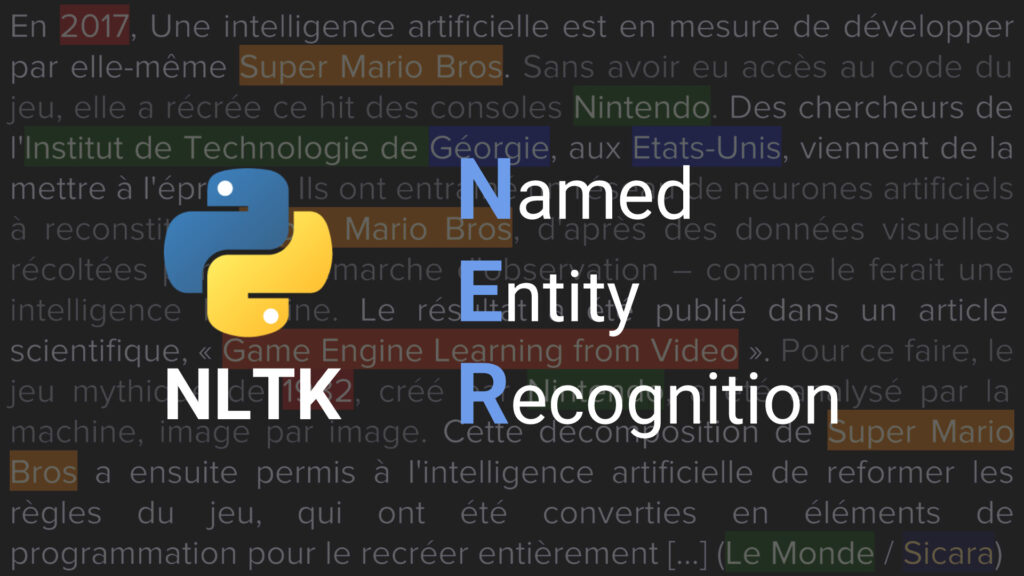
The classification report provides metrics such as precision, recall, and F1-score for each class. The confusion matrix further visualizes the model’s performance on individual classes.
In the final section, we’ll wrap up the blog post by summarizing the key points and highlighting potential areas for improvement.
Leave a Reply