Sets in Python are a versatile and powerful data type that provide a unique way to store and manipulate collections of elements. In this exploration, we will delve into the fascinating world of sets, understanding their creation, modification, and various operations that can be performed on them.
Creating Sets
A set is a collection of distinct and immutable elements. You can create a set by enclosing its elements in curly brackets {}
and separating them with commas. Additionally, the built-in set()
method can be used to create a set. Sets can include elements of various types such as integers, floats, tuples, and strings.
# Different types of sets in Python
# set of integers
Cogxta_Set = {1, 2, 3}
print(Cogxta_Set)
# set of mixed datatypes
Cogxta_Set = {1.0, "Python", (1, 2, 3)}
print(Cogxta_Set)
Output:
{1, 2, 3}
{1.0, (1, 2, 3), 'Python'}
It’s important to note that sets cannot have duplicate elements, and they do not support mutable items like lists, sets, or dictionaries.
Modifying Sets
Sets are mutable, allowing the addition and removal of elements. The add()
method adds a single element, while the update()
method can be used to add multiple elements, including those from lists and other sets.
# Modifying a set
Cogxta_Set = {1, 3}
Cogxta_Set.add(2)
Cogxta_Set.update([2, 3, 4])
Cogxta_Set.update([4, 5], {1, 6, 8})
print(Cogxta_Set)
Output:
{1, 2, 3, 4, 5, 6, 8}
Removing elements from a set can be achieved using methods like discard()
, remove()
, pop()
, and clear()
. These methods provide flexibility in managing set elements.
# Removing elements from a set
Cogxta_Set = {1, 3, 4, 5, 6}
Cogxta_Set.discard(4)
Cogxta_Set.remove(6)
Cogxta_Set.discard(2) # Element not present
Cogxta_Set.remove(4) # Element not present, raises an error
Cogxta_Set.pop() # Remove and return a random element
Cogxta_Set.clear() # Remove all elements
Set Operations
Sets support a variety of mathematical operations, such as union, intersection, difference, and symmetric difference. These operations can be performed using operators or methods.
# Set Operations
cog_A = {1, 2, 3, 4, 5}
cog_B = {4, 5, 6, 7, 8}
# Union
print(cog_A | cog_B) # Using operator
print(cog_A.union(cog_B)) # Using method
# Intersection
print(cog_A & cog_B) # Using operator
print(cog_A.intersection(cog_B)) # Using method
Output:
{1, 2, 3, 4, 5, 6, 7, 8} {1, 2, 3, 4, 5, 6, 7, 8} {4, 5} {4, 5}
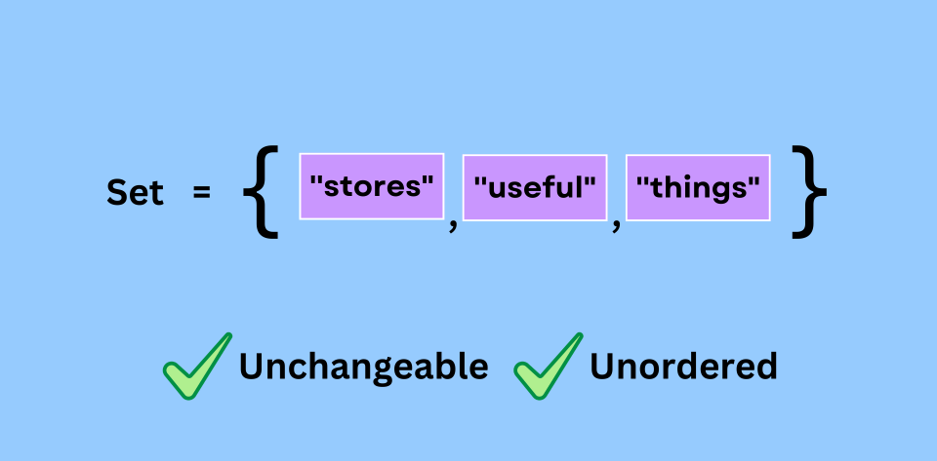
Set Methods
Python provides a set of built-in methods to manipulate sets. These methods offer functionalities like adding elements, removing elements, finding differences, and more.
add()
: Adds an element to the set.remove()
: Removes the specified element; raises an error if the element is not present.pop()
: Removes and returns a random element from the set.union()
: Returns a set containing the union of two sets.intersection()
: Returns a set that is the intersection of two sets.difference()
: Returns a set containing the difference between two sets.symmetric_difference()
: Returns a set with the symmetric differences of two sets.
These methods empower developers to perform a wide range of operations efficiently.
Conclusion
In conclusion, sets in Python are a valuable tool for handling collections of unique and immutable elements. Their mathematical operations, coupled with versatile methods, make them an essential part of the Python programming landscape. Whether you’re dealing with data manipulation, removing duplicates, or performing set operations, sets provide an elegant and efficient solution to various programming challenges.
Leave a Reply